A method destructured from an object loses its original context
My first of (hopefully) many stories where I expose how little I know about coding and share the lessons I learned (or re-learned).
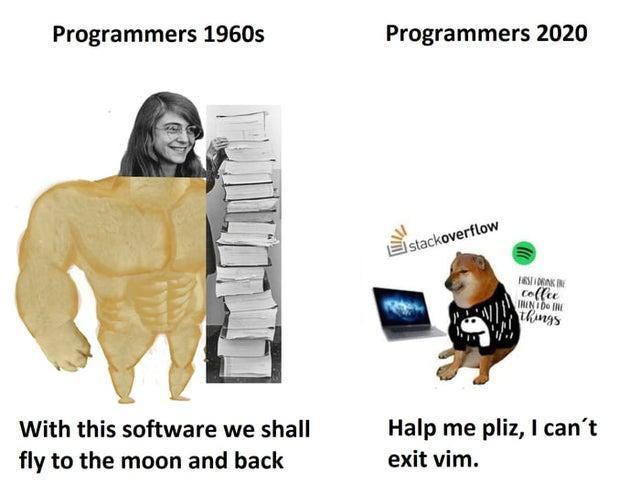
When defining a method on an object, we presumably want this
to refer to the object on which the method is defined.
const someObj = {
someProp: 'someValue',
someMethod: function() {
console.log(this.someProp);
}
};someObj.someMethod(); // will log 'someValue'
Since all the cool kids do it, I use destructuring assignments all over the place. However, destructuring a method from an object causes it to lose its original context. In other words, when invoking a destructured method, this
no longer points to the object on which the method is defined.
const someObj = {
someProp: 'someValue',
someMethod: function() {
console.log(this.someProp);
}
};const { someMethod } = someObj;someMethod(); // will log 'undefined'
Similarly, assigning a method defined on an object to a new variable, and invoking that new function variable causes it to lose its original context as well. In fact, Babel transpiles the code snippet above into the code snippet below to support the one and only browser that doesnβt support destructuring assignments.
const someObj = {
someProp: 'someValue',
someMethod: function() {
console.log(this.someProp);
}
};const someFunction = someObj.someMethod;someFunction(); // will log 'undefined'
Today I (re)learned.